前回のおさらい
チャートを作成した
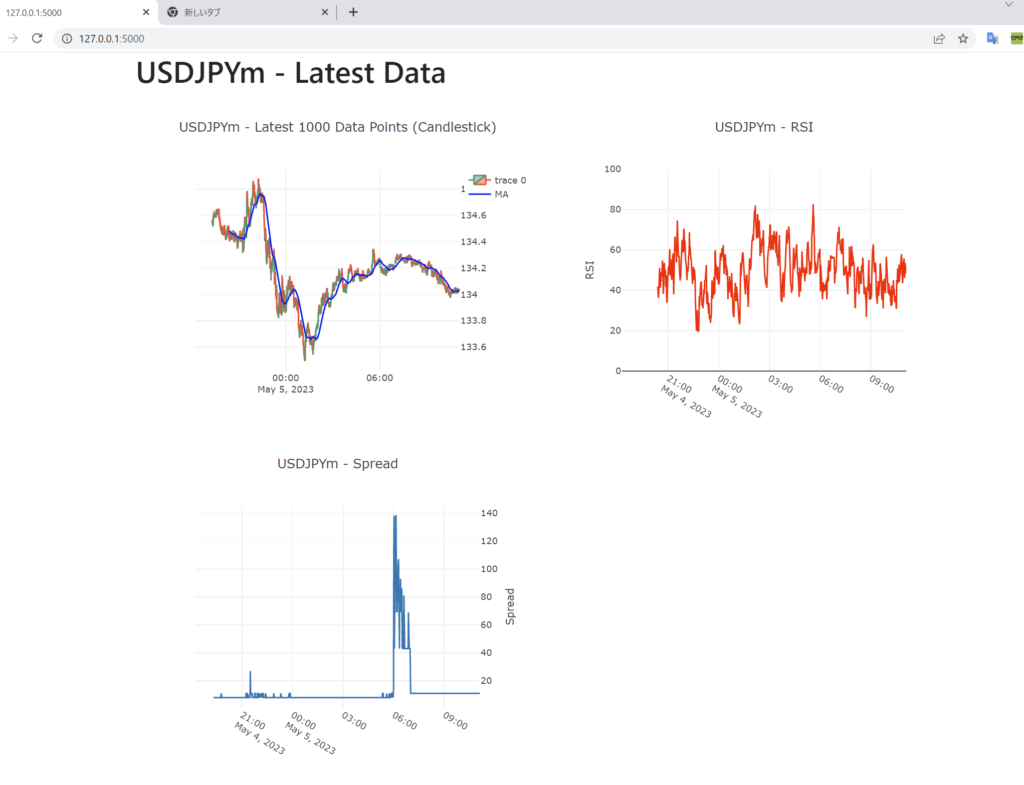
Table of Contents
チャート機能を消す
Project
├── mt5_price_set_DB.py
├── requirements.txt
└── technical_set_DB.py
せっかくですが、チャートを表示する機能は消しました。必要な機能だけあればいい。チャートはいらなかったんや。
今のこった機能は、
- MT5からデータを取得する機能
- テクニカル指標を計算する機能
です。
前回はチャートでデータを確認したので今回はデータベースのテーブルを確認することメインにやっていきます。
まずはtest.pyを作ります。
price_dataテーブルをターミナルでprintして確認する
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import sqlite3 DB_NAME = "price_data.db" TABLE_NAME = "price_data" # SQLite3 connection conn = sqlite3.connect(DB_NAME) c = conn.cursor() # Fetch the latest 100 rows c.execute(f"SELECT * FROM {TABLE_NAME} ORDER BY id DESC LIMIT 100") rows = c.fetchall() # Print the data for row in rows: print(row) |
うん。めっちゃシンプルですね。
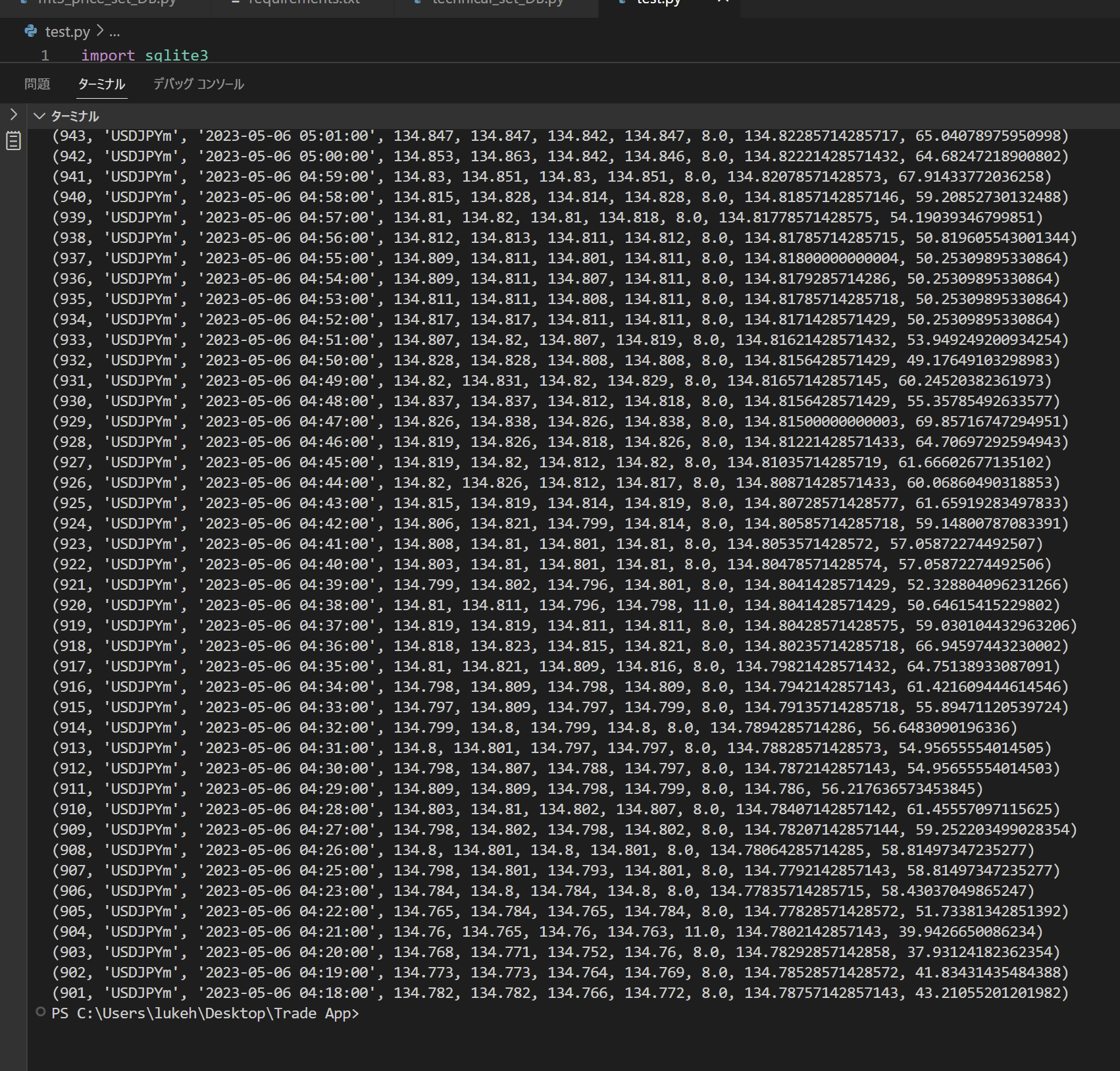
実行させるとデータベースから取得データが値だけ表示されます。
ブラウザでデータベースの中身を確認する
テキストだけだと見づらい場合はflaskでブラウザで確認できるようにしよう。templatesファルダを作成して、中にindex.htmlを作る。
.
├── templates
│ └── index.html
├── mt5_price_set_DB.py
├── price_data.db
├── requirements.txt
├── technical_set_DB.py
└── test.py
templates
: HTMLテンプレートを格納するディレクトリ。mt5_price_set_DB.py
: MT5に接続して価格データを取得し、SQLiteデータベースに保存するためのスクリプト。price_data.db
: SQLiteデータベースのファイル。requirements.txt
: Pythonパッケージの依存関係を記述したファイル。technical_set_DB.py
: SQLiteデータベースから価格データを取得し、テクニカル指標を計算して更新するためのスクリプト。test.py
: Flaskアプリケーションを起動するためのスクリプト。
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
<!doctype html> <html> <head> <title>Price Data</title> </head> <body> <h1>Price Data</h1> <table> <tr> <th>ID</th> <th>Symbol</th> <th>Time</th> <th>Open</th> <th>High</th> <th>Low</th> <th>Close</th> <th>Max Spread</th> </tr> {% for row in rows %} <tr> <td>{{ row[0] }}</td> <td>{{ row[1] }}</td> <td>{{ row[2] }}</td> <td>{{ row[3] }}</td> <td>{{ row[4] }}</td> <td>{{ row[5] }}</td> <td>{{ row[6] }}</td> <td>{{ row[7] }}</td> </tr> {% endfor %} </table> </body> </html> |
test.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
from flask import Flask, render_template import sqlite3 DB_NAME = "price_data.db" TABLE_NAME = "price_data" app = Flask(__name__) @app.route('/') def index(): # SQLite3 connection conn = sqlite3.connect(DB_NAME) c = conn.cursor() # Fetch the latest 100 rows c.execute(f"SELECT * FROM {TABLE_NAME} ORDER BY id DESC LIMIT 100") rows = c.fetchall() # Close the connection conn.close() # Render the HTML template with the rows return render_template('index.html', rows=rows) if __name__ == '__main__': app.run() |
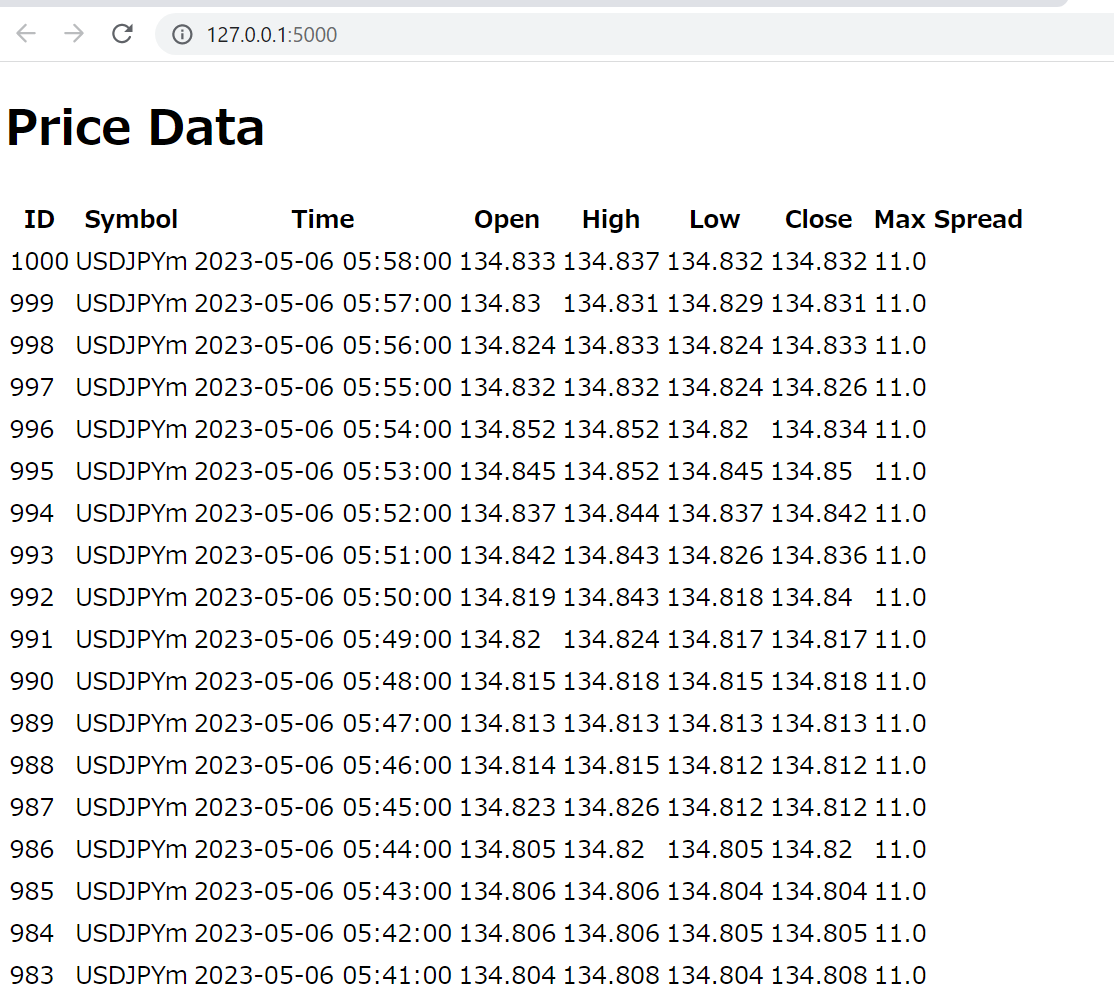
さっきと比べてカラム名が確認しやすいね。CSSで見た目を成形しよう。
ブラウザでデータベースの中身を確認するデザイン調整
CSSでデザインの変更とRSIが30以下、70以上なら色を変えるように変更してみる。
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
<!doctype html> <html> <head> <title>Price Data</title> <!-- Bootstrap CSS --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous"> </head> <body> <div class="container"> <h1>Price Data</h1> <table class="table"> <thead> <tr> <th>ID</th> <th>Symbol</th> <th>Time</th> <th>Open</th> <th>High</th> <th>Low</th> <th>Close</th> <th>Max Spread</th> <th>MA</th> <th>RSI</th> </tr> </thead> <tbody> {% for row in rows %} <tr> <td>{{ row[0] }}</td> <td>{{ row[1] }}</td> <td>{{ row[2] }}</td> <td>{{ row[3] }}</td> <td>{{ row[4] }}</td> <td>{{ row[5] }}</td> <td>{{ row[6] }}</td> <td>{{ row[7] }}</td> <td>{{ row[8] }}</td> <td class="{% if row[9] is not none and row[9] <= 30 %}bg-danger text-white font-weight-bold{% elif row[9] is not none and row[9] >= 70 %}bg-primary text-white font-weight-bold{% endif %}">{{ row[9] }}</td> </tr> {% endfor %} </tbody> </table> </div> <!-- Bootstrap JS --> <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script> </body> </html> |
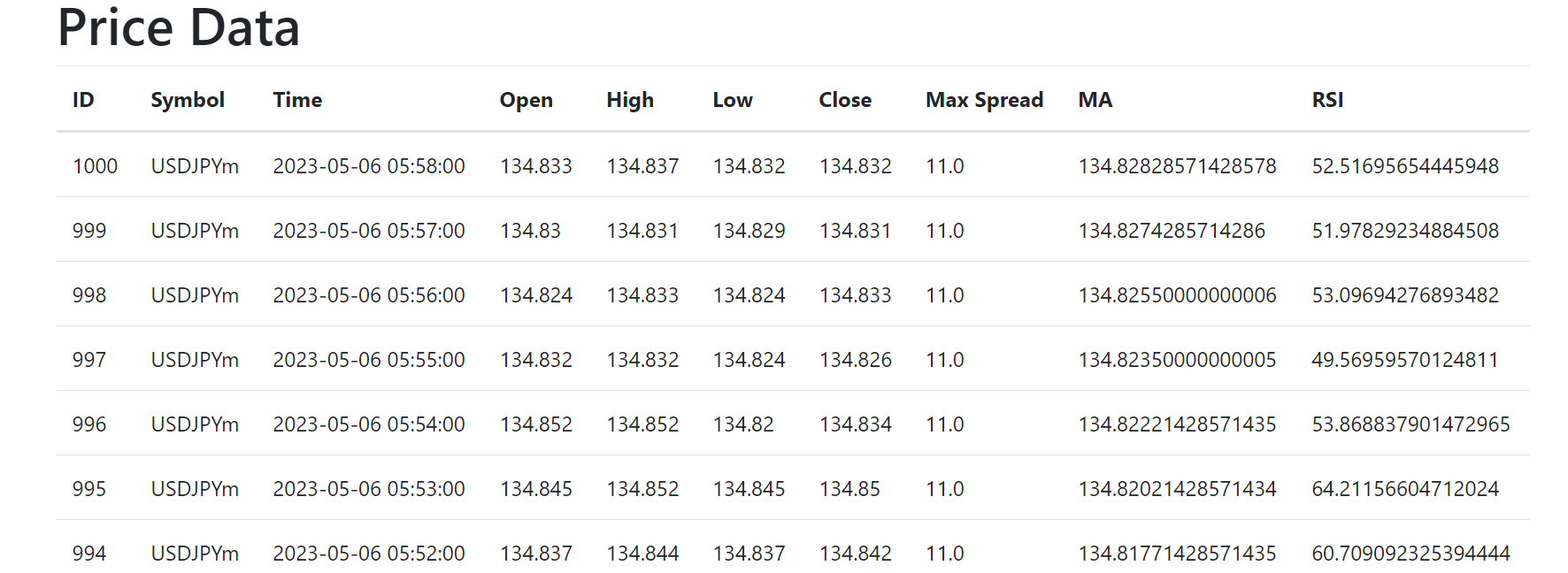
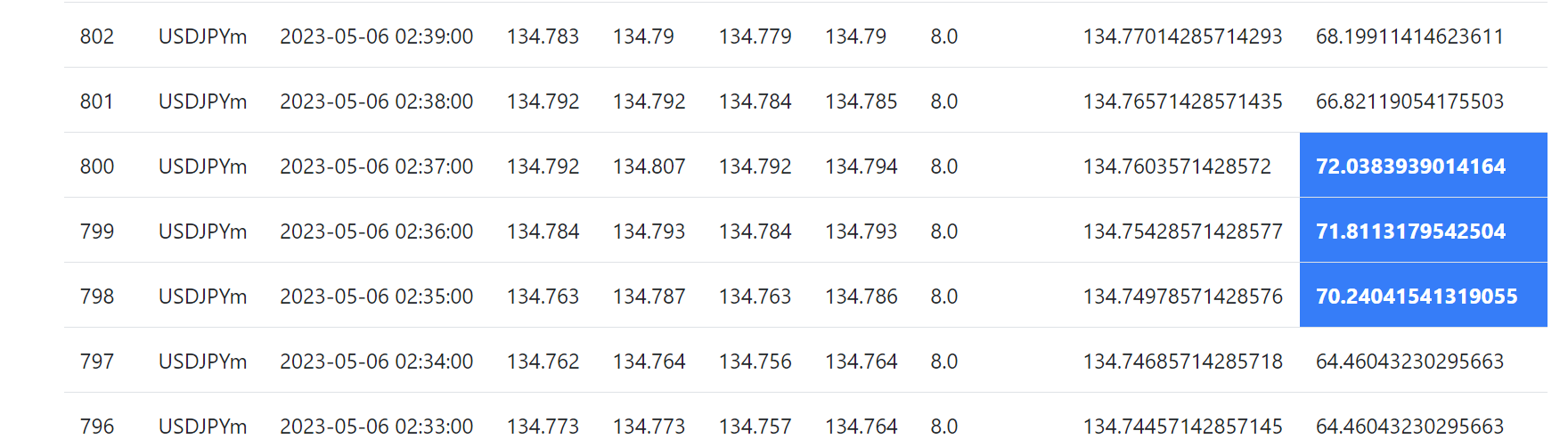
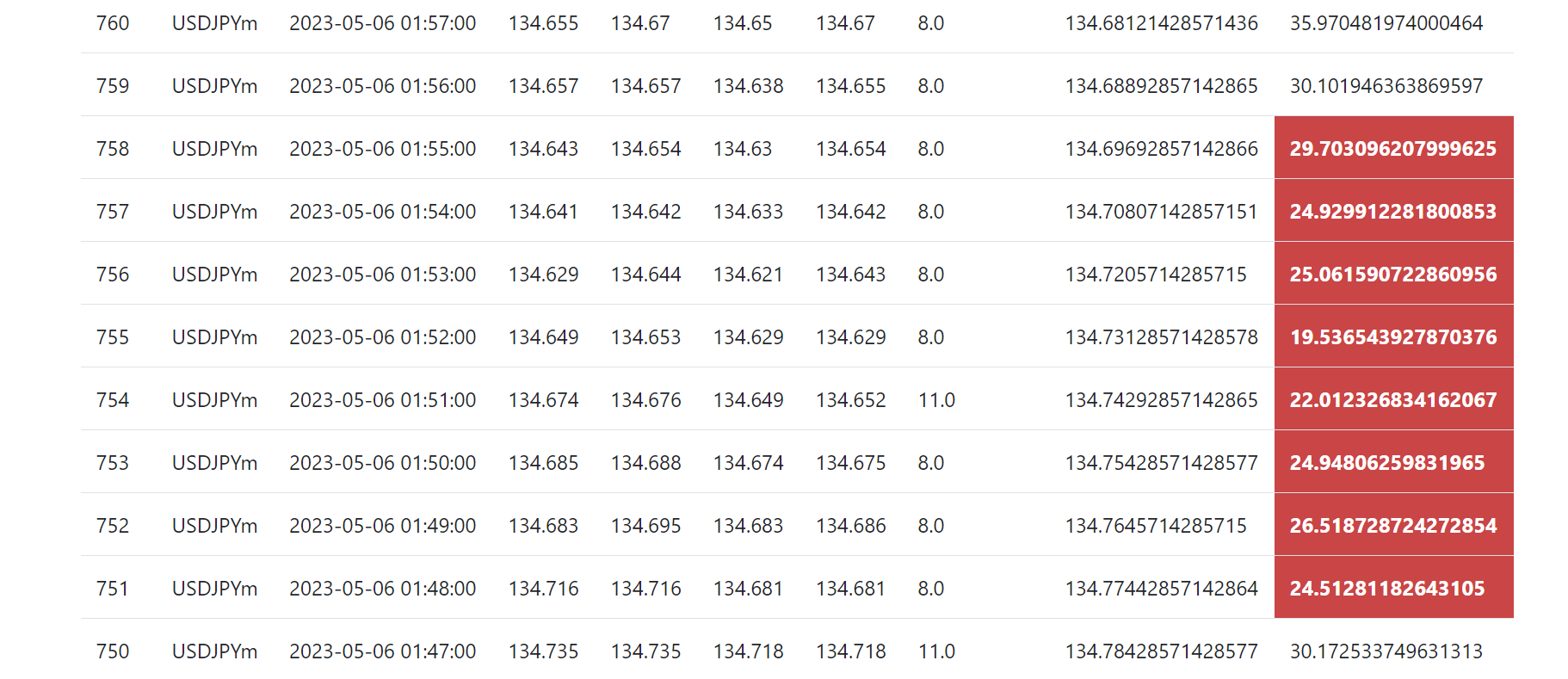
みやすい。
pandasで確認する
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import sqlite3 import pandas as pd DB_NAME = "price_data.db" TABLE_NAME = "price_data" # SQLite3 connection conn = sqlite3.connect(DB_NAME) # Fetch the latest 100 rows and convert to DataFrame df = pd.read_sql_query(f"SELECT * FROM {TABLE_NAME} ORDER BY id DESC LIMIT 100", conn) # Print the data print(df) |
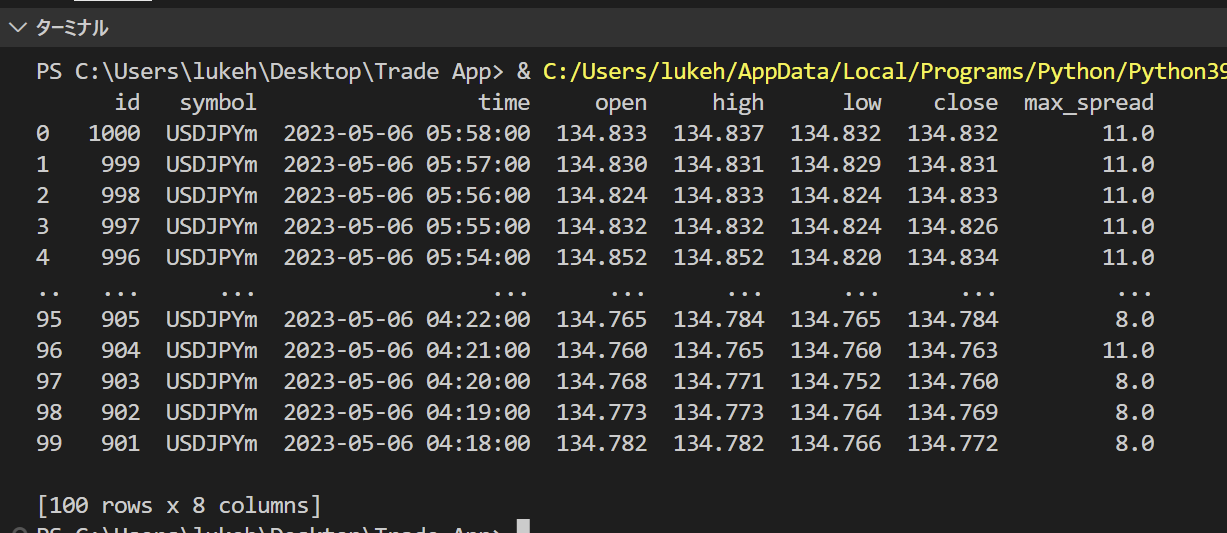
超簡単
データベースのテーブルの確認おわり。
BybitがGoogleのIPアドレス規制をしているためです。国内のVPSなら使…
自分のbotで使ってるAPIキーを使用しているんですが、 You have br…
pybit 最新版にコードを変更しました。コードとrequirements.tx…
お返事ありがとうございます。はい。pybit==2.3.0になっております。
コードはあっていると思います。rewuirements.txtは「pybit==…